Scrapy是Python优秀的爬虫框架,selenium是非常好用的自动化WEB测试工具,两者结合可以非常容易对动态网页进行爬虫。
本文的需求是抓取UC头条各个板块的内容。UC头条(https://news.uc.cn/ )网站没有提供搜索入口,只能每个板块的首页向下滚动鼠标加载更多。要对这样的网站进行检索,抓取其内容,采用一般的scrapy请求方式,每次只能获取最新的10条数据,分析其JS请求,发现参数过于复杂,没有规律。如果想获取更多数据,则需要采用模拟浏览器的方法,这时候selenium就派上用场了。
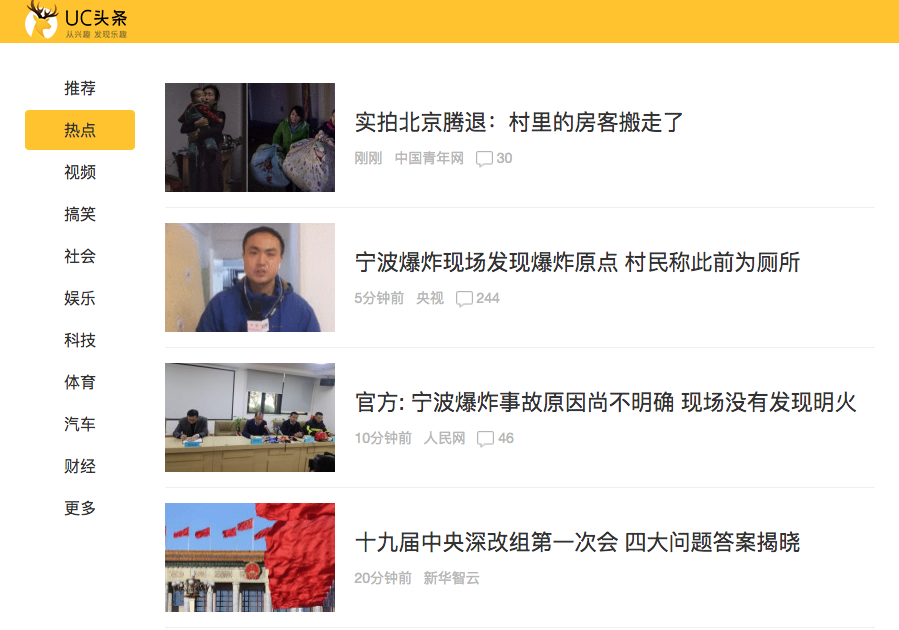
1,定义spider
模拟从百度搜索进入,这个步骤可以省略,主要为了跳到parse函数
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| class UCTouTiaoSpider(VideoBaseSpider): name = "uctoutiao_spider" df_keys = ['人物', '百科', '乌镇'] def __init__(self, scrapy_task_id=None, *args, **kwargs): self.url_src = "http://www.baidu.com" def start_requests(self): requests = [] request = scrapy.Request("http://www.baidu.com", callback=self.parse) requests.append(request) return requests
|
2,parse函数
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55
| def parse(self, response): self.log(response.url) urls = ["https://news.uc.cn/", "https://news.uc.cn/c_redian/", # "https://news.uc.cn/c_shipin/", # "https://news.uc.cn/c_gaoxiao/", "https://news.uc.cn/c_shehui/", "https://news.uc.cn/c_yule/", "https://news.uc.cn/c_keji/", "https://news.uc.cn/c_tiyu/", "https://news.uc.cn/c_qiche/", "https://news.uc.cn/c_caijing/", "https://news.uc.cn/c_junshi/", "https://news.uc.cn/c_tansuo/", "https://news.uc.cn/c_lishi/", "https://news.uc.cn/c_youxi/", "https://news.uc.cn/c_lvyou/", "https://news.uc.cn/news/", "https://news.uc.cn/c_shishang/", "https://news.uc.cn/c_jiankang/", "https://news.uc.cn/c_guoji/", "https://news.uc.cn/c_yuer/", "https://news.uc.cn/c_meishi/"] # 启动浏览器,这里用的火狐,如果在linux环境下可以用PhantomJS,稳定性稍微差点,有内存泄露的风险。 driver = webdriver.Firefox() for url in urls: try: print(url) driver.get(url) #模拟鼠标滚到底部(加载100条数据) for _ in range(10): driver.execute_script("window.scrollTo(0, document.body.scrollHeight)") driver.implicitly_wait(10) # 隐性等待,最长10秒 # print driver.page_source soup = bs(driver.page_source, 'lxml') articles = soup.find_all(href=re.compile("/a_\w+?/"), text=re.compile(".+")) for article in articles: for key in self.df_keys: item = VideoItem() #自定义的Item item['title'] = article.text item['href'] = article['href'] self.log(item) yield item except Exception as e: print e if driver == None: driver = webdriver.Firefox() if driver != None: driver.quit()
|
真正的实现部分比较简单,几句代码就搞定了。
附:
selenium使用实例
1,切换焦点至新窗口
在页面上点击一个button, 然后打开了一个新的window, 将当前IWebDriver的focus切换到新window,使用IWebDriver.SwitchTo().Window(string windowName)。
例如, 我点击按钮以后弹出一个名字叫做”Content Display”的window, 要切换焦点到新窗口的方法是, 首先,获得新window的window name, 大家不要误以为page tile就是window name 哦, 如果你使用driver.SwitchTo().Window(“Content Display”)是找不到window name 叫做”Content Display”的窗口的, 其实Window Name 是一长串数字,类似“59790103-4e06-4433-97a9-b6e519a84fd0”。
要正确切换到”Content Display”的方法是:
获得当前所有的WindowHandles。
循环遍历到所有的window, 查找window.title与”Content Display”相符的window返回。
1 2 3 4 5
| for handle in dr.window_handles: dr.switch_to.window(handle) print dr.title if len(dr.title) == '目标窗口标题': break
|
参考:Selenium - IWebDriver.SwitchTo() frame 和 Window 的用法
2 ,移至底部
1
| driver.execute_script("window.scrollTo(0, document.body.scrollHeight)")
|
3,移动至指定元素
某些按钮点击时必须可见,于是要把屏幕移动到按钮可见的区域
1 2 3 4 5 6 7 8
| element = driver.find_element_by_xpath("//a[@class='p-next']") element.location_once_scrolled_into_view #或者 driver.set_window_size(800,800) element = driver.find_element_by_xpath("//a[@class='p-next']") js = "window.scrollTo({},{});".format(element.location['x'], element.location['y'] - 100) driver.execute_script(js)
|
参考:
Python selenium —— 一定要会用selenium的等待,三种等待方式解读